Interactive Web Pages with jQuery: Essential Techniques
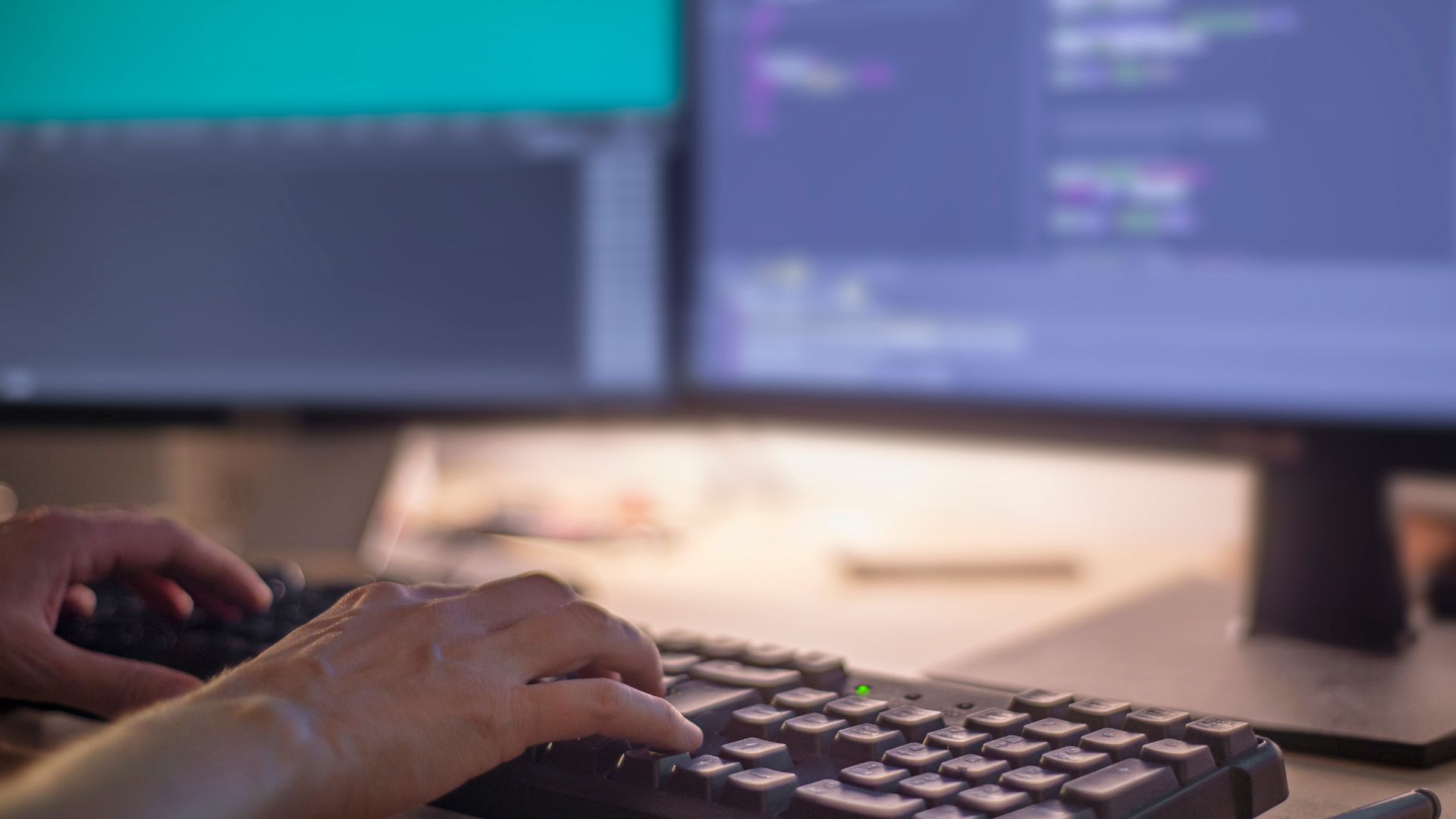
jQuery, a fast and concise JavaScript library, simplifies HTML document traversal, event handling, animating, and Ajax interactions for rapid web development. This guide covers essential techniques for making web pages interactive with jQuery.
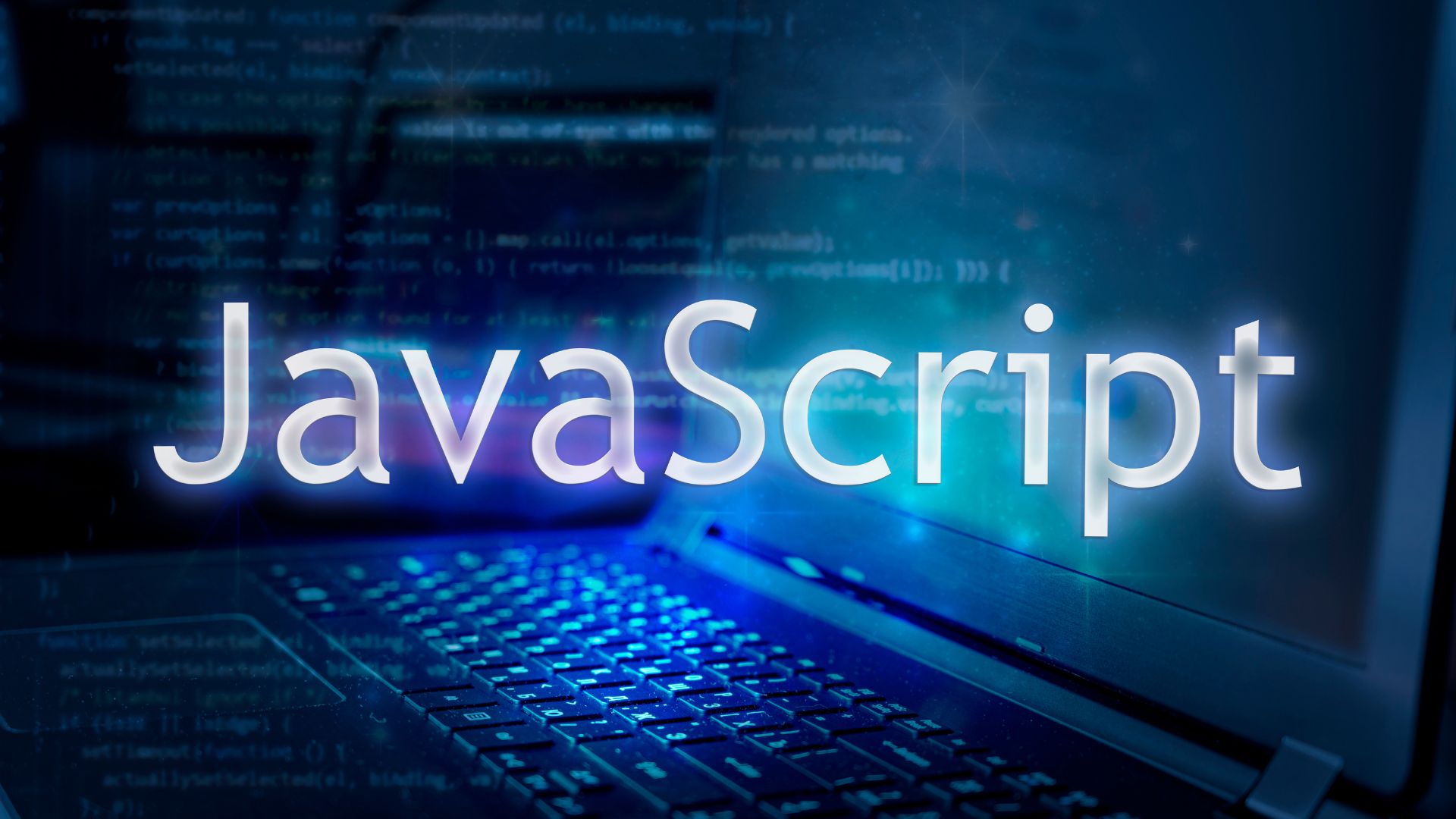
1. Understanding jQuery Basics
- Query Syntax: The jQuery syntax is designed to select HTML elements and perform some action on them. A basic syntax looks like `$(selector).action()`, where `$` accesses jQuery, `selector` finds HTML elements, and `action()` is performed on the element(s).
- Document Ready Event: Before you can safely manipulate a webpage using jQuery, you need to ensure the document is fully loaded. This is done using `$(document).ready()`.
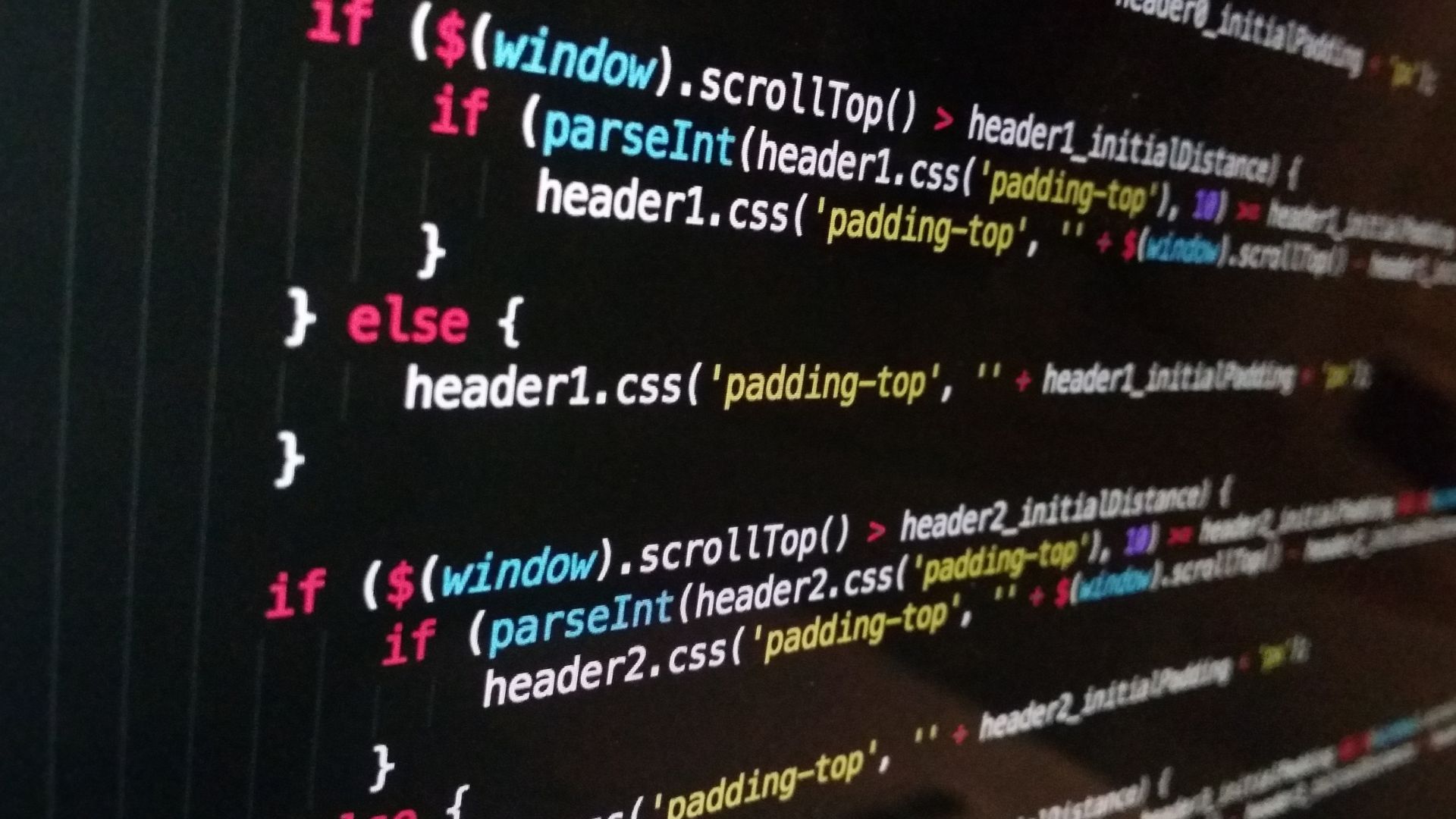
2. Selecting Elements
jQuery's powerful selection capabilities allow you to target HTML elements in various ways, such as by tag name, class, id, or even more complex CSSstyle selectors. For example, `$('.classname')` selects all elements with the specified class.
3. Manipulating the DOM
- Changing Content: Methods like `.text()`, `.html()`, and `.val()` are used to get or set the textual content, HTML content, and value of form fields, respectively.
- Changing CSS: jQuery's `.css()` method lets you get or set the style of elements. You can change multiple CSS properties at once.
4. Handling Events
jQuery simplifies event handling. Whether it’s a click, hover, or keypress event, you can easily bind these events to elements. For example, `$('#element').click(function(){ /* action */ })` binds a click event.
5. Animations and Effects
jQuery comes with several builtin effects and animations, like `.hide()`, `.show()`, `.fadeIn()`, `.fadeOut()`, `.slideUp()`, `.slideDown()`, and more. You can also create custom animations using the `.animate()` method.
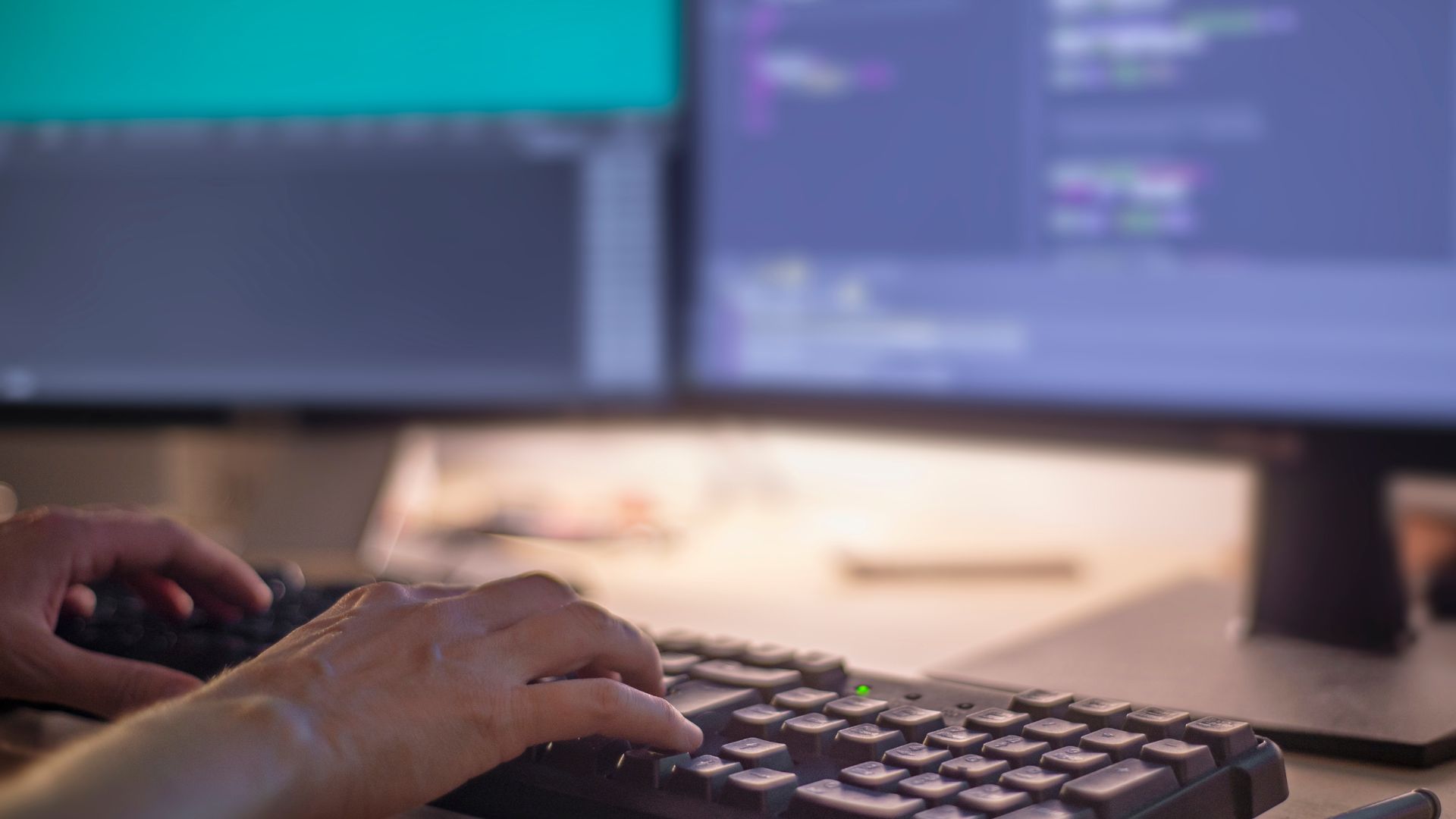
6. AJAX with jQuery
With jQuery, making AJAX calls becomes straightforward, allowing you to load data asynchronously without a page refresh. Methods like `$.ajax()`, `$.get()`, and `$.post()` are used for AJAX requests.
7. jQuery UI for Enhanced Interactivity
jQuery UI is a collection of GUI widgets, animated visual effects, and themes implemented with jQuery, CSS, and HTML. This can be used to add more complex components like date pickers, sliders, and dialog boxes.
8. Best Practices
- Keep jQuery Up to Date: Always use the latest version for more features and security.
- Unobtrusive JavaScript: Keep content, design, and behavior separate. Avoid inline JavaScript.
- Performance Considerations: Be mindful of selectors’ performance and avoid complex selectors.
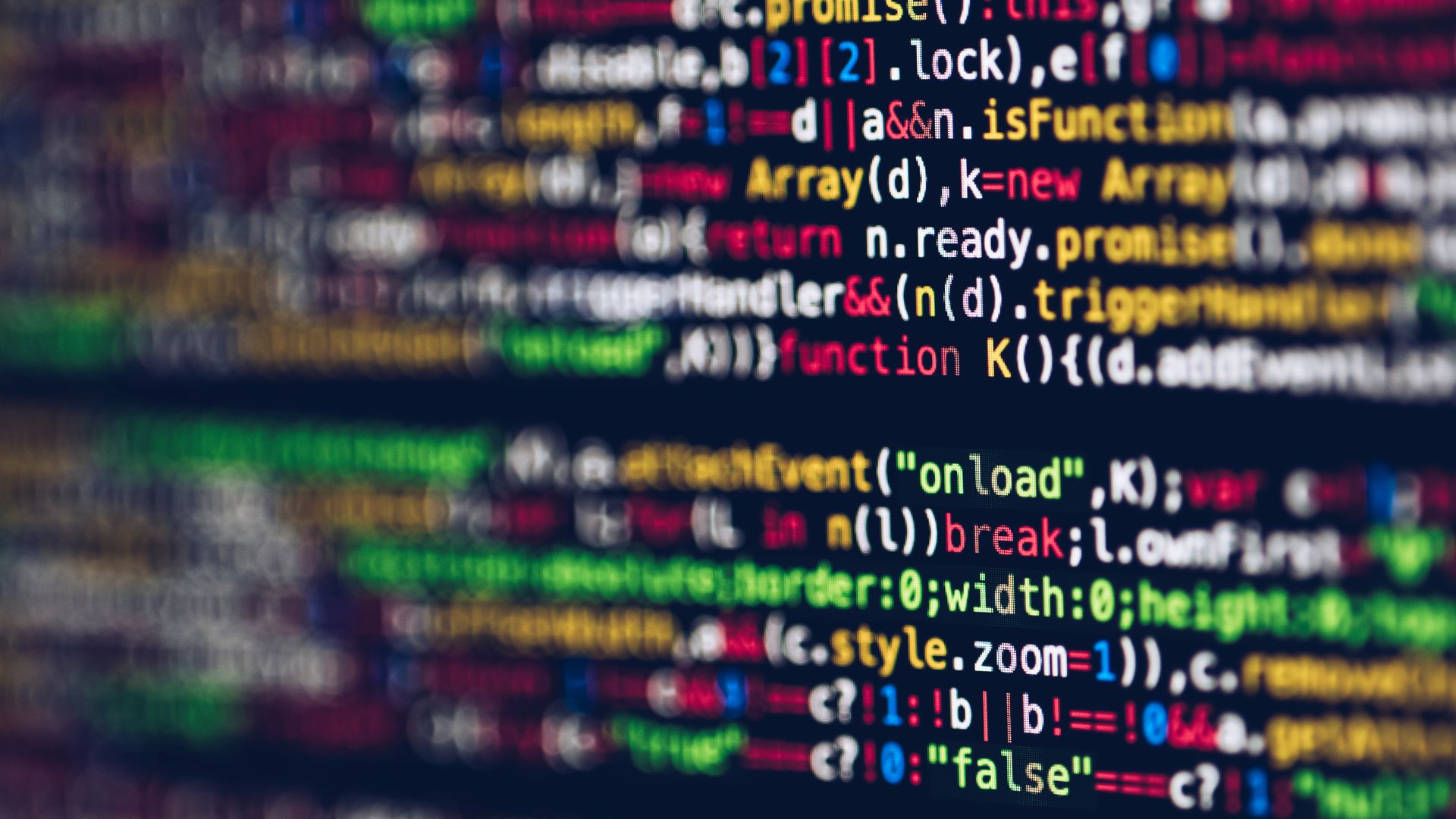
jQuery remains a popular tool for web developers, thanks to its simplicity, crossbrowser compatibility, and extensibility. By mastering jQuery, you can add a level of interactivity and polish to your web pages that enhances user experience significantly. Whether you are a beginner or an experienced web developer, jQuery offers a range of functionalities that can streamline the process of making a dynamic and responsive web application.